출처 : https://blog.bitsrc.io/6-tricks-with-resting-and-spreading-javascript-objects-68d585bdc83
7 Tricks with Resting and Spreading JavaScript Objects
Resting and spreading can be used for more than just resting arguments and spreading arrays.
blog.bitsrc.io
7 Tricks with Resting and Spreading JavaScript Objects
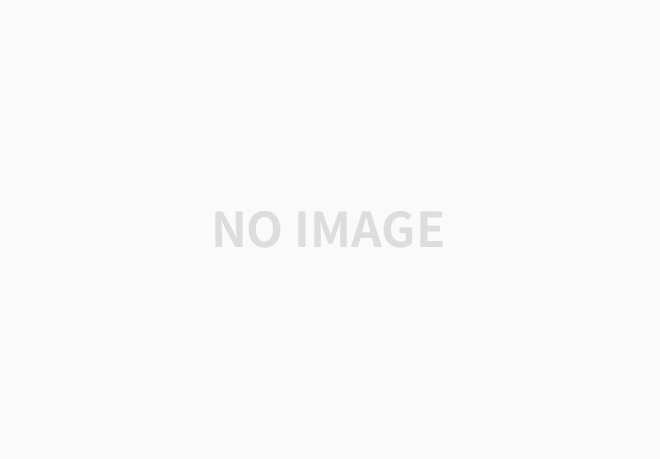
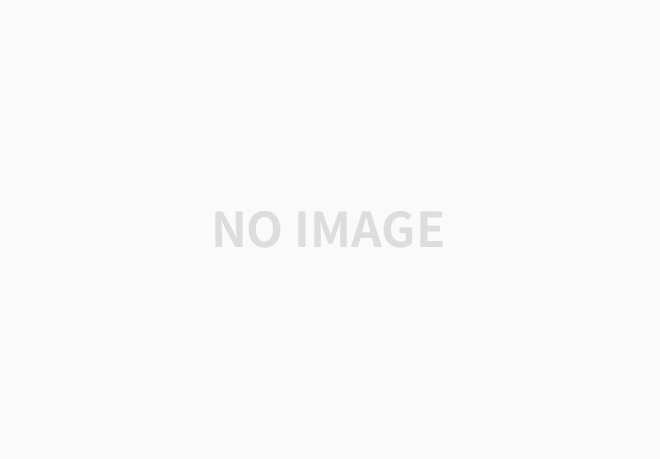
Resting and spreading can be used for more than just resting arguments and spreading arrays.
Here are six lesser known tricks when using rest and spread with JavaScript objects.
1. Adding Properties
Clone an object while simultaneously adding additional properties to the (shallow) cloned object.
In this example user is cloned and password is added into userWithPass.
const user = { id: 100, name: 'Howard Moon'}
const userWithPass = { ...user, password: 'Password!' }
user //=> { id: 100, name: 'Howard Moon' }
userWithPass //=> { id: 100, name: 'Howard Moon', password: 'Password!' }
2. Merge Objects
Merge two objects into a new object.
part1 and part2 are merged together into user1.
const part1 = { id: 100, name: 'Howard Moon' }
const part2 = { id: 100, password: 'Password!' }
const user1 = { ...part1, ...part2 }
//=> { id: 100, name: 'Howard Moon', password: 'Password!' }
Objects also be merged with this syntax:
const partial = { id: 100, name: 'Howard Moon' }
const user = { ...partial, id: 100, password: 'Password!' }
user //=> { id: 100, name: 'Howard Moon', password: 'Password!' }
3. Exclude Object Properties
Properties can be removed using destructuring in combination with the rest operator. Here password is destructured out (ignored) and the rest of the properties are returned as rest.
const noPassword = ({ password, ...rest }) => rest
const user = {
id: 100,
name: 'Howard Moon',
password: 'Password!'
}
noPassword(user) //=> { id: 100, name: 'Howard moon' }
4. Dynamically Exclude Properties
The removeProperty function takes a prop as an argument. Using computed object property names the prop can be excluded dynamically from the clone.
const user1 = {
id: 100,
name: 'Howard Moon',
password: 'Password!'
}
const removeProperty = prop => ({ [prop]: _, ...rest }) => rest
// ---- ------
// \ /
// dynamic destructuring
const removePassword = removeProperty('password')
const removeId = removeProperty('id')
removePassword(user1) //=> { id: 100, name: 'Howard Moon' }
removeId(user1) //=> { name: 'Howard Moon', password: 'Password!' }
5. Organize Properties
Sometimes properties aren’t in the order we need them to be. Using a couple of tricks we can push properties to the top of the list or move them to the bottom.
To move id to the first position, add id: undefined to the new Object before spreading object.
const user3 = {
password: 'Password!',
name: 'Naboo',
id: 300
}
const organize = object => ({ id: undefined, ...object })
// -------------
// /
// move id to the first property
organize(user3)
//=> { id: 300, password: 'Password!', name: 'Naboo' }
To move password to the last property, first destructe password out of object. Then set password after spreading object.
const user3 = {
password: 'Password!',
name: 'Naboo',
id: 300
}
const organize = ({ password, ...object }) =>
({ ...object, password })
// --------
// /
// move password to last property
organize(user3)
//=> { name: 'Naboo', id: 300, password: 'Password!' }
6. Default Properties
Default properties are values that will be set only when they are not included in the original object.
In this example, user2 does not contain quotes. The setDefaults function ensures all objects have quotes set otherwise it will be set to [].
When calling setDefaults(user2), the return value will include quotes: [].
When calling setDefaults(user4), because user4 already has quotes, that property will not be modified.
const user2 = {
id: 200,
name: 'Vince Noir'
}
const user4 = {
id: 400,
name: 'Bollo',
quotes: ["I've got a bad feeling about this..."]
}
const setDefaults = ({ quotes = [], ...object}) =>
({ ...object, quotes })
setDefaults(user2)
//=> { id: 200, name: 'Vince Noir', quotes: [] }
setDefaults(user4)
//=> {
//=> id: 400,
//=> name: 'Bollo',
//=> quotes: ["I've got a bad feeling about this..."]
//=> }
It can also be written like this if you want the defaults to appear first instead of last:
const setDefaults = ({ ...object}) => ({ quotes: [], ...object })
7: Renaming Properties
By combining the techniques above, a function can be created to rename properties.
Imagine there are some objects with an uppercase ID that should be lowercase. Start by destructuring ID out of the object. Then add it back as id while object is being spread.
const renamed = ({ ID, ...object }) => ({ id: ID, ...object })
const user = {
ID: 500,
name: "Bob Fossil"
}
renamed(user) //=> { id: 500, name: 'Bob Fossil' }
8. Bonus! Add conditional properties
Thanks for @vinialbano for pointing out you can also conditionally add properties. In this example, password will be added only when password is truthy!
const user = { id: 100, name: 'Howard Moon' }
const password = 'Password!'
const userWithPassword = {
...user,
id: 100,
...(password && { password })
}
userWithPassword //=> { id: 100, name: 'Howard Moon', password: 'Password!' }
Summary
I tried to list out a handful lesser known spread and rest tricks, if you know any that I haven’t listed here, please let everyone know in the comments! If you learned something new, please share on Twitter and with your friends, it really helps!
Follow me here or on Twitter @joelnet!
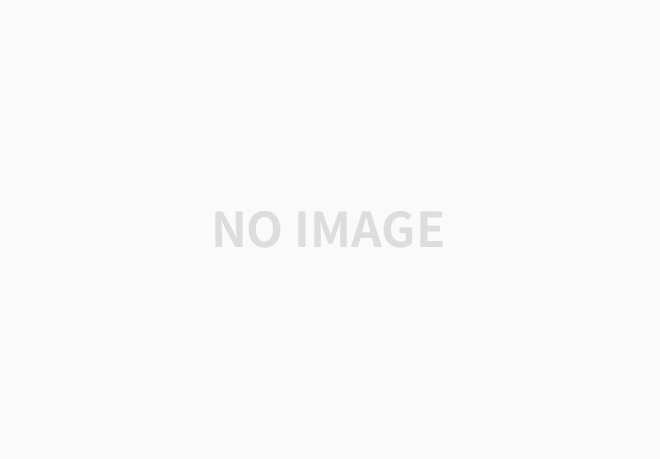
Cheers!
Learn more
The Chronicles of JavaScript Objects
Everything you need to know: The Operations, Properties and the Prototype
blog.bitsrc.io
https://blog.bitsrc.io/the-chronicles-of-javascript-objects-2d6b9205cd66
The Chronicles of JavaScript Objects
Everything you need to know: The Operations, Properties and the Prototype
blog.bitsrc.io
Diving Deeper in JavaScripts Objects
A Closer Look at JavaScript Object Descriptors
blog.bitsrc.io
https://blog.bitsrc.io/diving-deeper-in-javascripts-objects-318b1e13dc12
Diving Deeper in JavaScripts Objects
A Closer Look at JavaScript Object Descriptors
blog.bitsrc.io
5 Tools for Faster Development in React
5 tools to speed the development of your React application, focusing on components.
blog.bitsrc.io
https://blog.bitsrc.io/5-tools-for-faster-development-in-react-676f134050f2
5 Tools for Faster Development in React
5 tools to speed the development of your React application, focusing on components.
blog.bitsrc.io
Tip: Use Bit to easily discover and share components between apps. Build multiple projects faster with shared code and share your components. Try it.
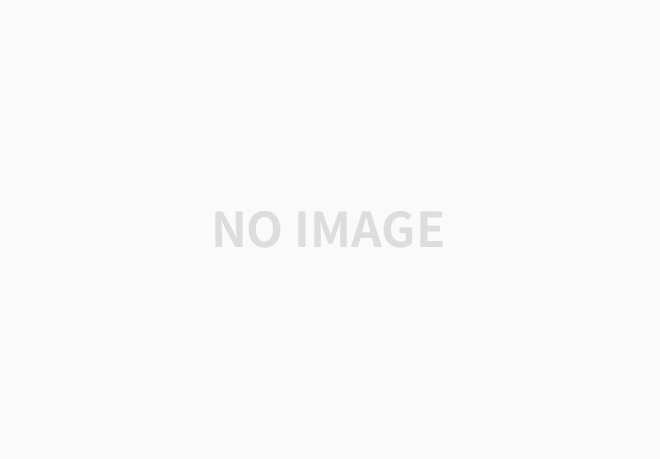
Component Discovery and Collaboration · Bit
Bit is where developers share components and collaborate to build amazing software together. Discover components shared…
bit.dev
https://learnjs.vlpt.us/useful/06-destructuring.html
06. 비구조화 할당 (구조 분해) 문법 · GitBook
06. 비구조화 할당 (구조분해) 문법 이번에는 1장 섹션 6 에서도 배웠던 비구조화 할당 문법을 잘 활용하는 방법에 대해서 알아보겠습니다. 이전에 배웠던것을 복습해보자면, 비구조화 할당 문법
learnjs.vlpt.us
06. 객체 · GitBook
06. 객체 객체는 우리가 변수 혹은 상수를 사용하게 될 때 하나의 이름에 여러 종류의 값을 넣을 수 있게 해줍니다. const dog = { name: '멍멍이', age: 2 }; console.log(dog.name); console.log(dog.age); 결과물은 다
learnjs.vlpt.us
'Web > Javascript|TypeScript' 카테고리의 다른 글
[TS] typescript-excess-property-checks (0) | 2021.08.31 |
---|---|
[classnames] Could not find a declaration file for module (0) | 2021.07.07 |
[JAVASCRIPT] 한번 쯤 궁금한것 (0) | 2021.06.30 |
[Javascript] 입문/초보 참고 사이트 (0) | 2021.06.22 |
[JAVASCRIPT] Navigator (위도, 경도) (0) | 2021.05.25 |